Type or Interface For Typescript Projects ?
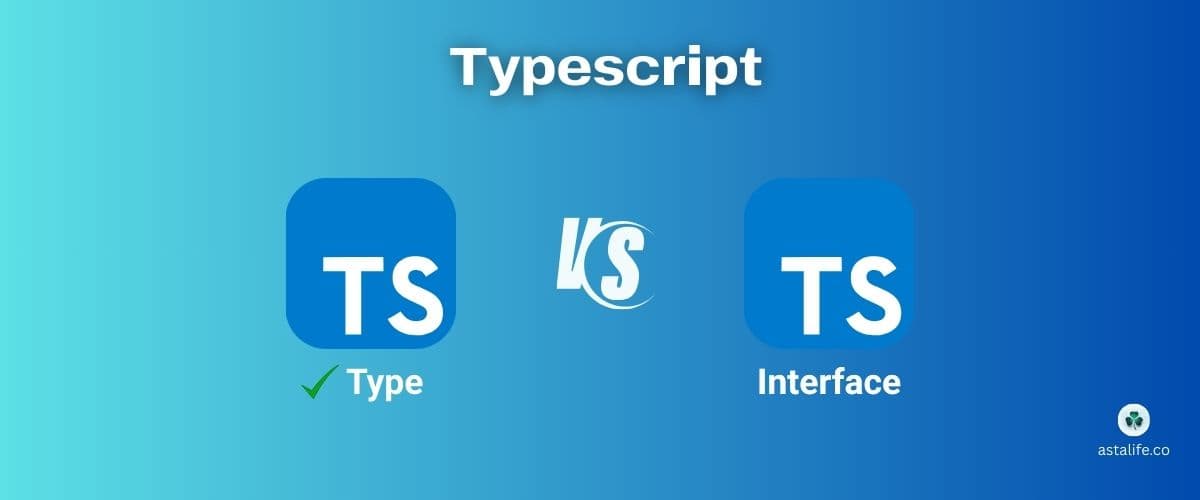
Discover why I prefer type over interface in TypeScript! This post dives into its flexibility and practical advantages, helping you write cleaner, more efficient code. 🚀
Why You Should Use Type Instead of Interface in TypeScript
TypeScript provides two main ways to define the shape of an object: type and interface. While both can be used interchangeably in many cases, there are specific scenarios where type offers more flexibility and power. This blog will explore these scenarios and explain why you might prefer type over interface.
Key Differences:
1. Union Types
type can define union types, which an interface cannot.
1type Result = Success | Failure;
2. Intersection Types
type can define intersection types.
1type Combined = TypeA & TypeB;
3. Primitive Types
type can alias primitive types.
1type StringAlias = string;
4. Tuples
type can define tuples
1type Tuple = [number, string];
5. Mapped Types
type can create mapped types
1type Readonly = { readonly [P in keyof T]: T[P]; };
6. Complex Type Constructs
type can define more complex type constructs like conditional types.
1type Conditional = T extends string ? string : number;
Let's consider a React component where we define the props using type instead of interface.
7. Union Types in React component
1// Case type
2type ButtonProps = {
3 label: string;
4 onClick: () => void;
5 variant: 'primary' | 'secondary';
6}
7
8const Button: React.FC<ButtonProps> = ({ label, onClick, variant }) => {
9 return (
10 <button
11 className={`btn-${variant}`}
12 onClick={onClick}
13 >
14 {label}
15 </button>
16 );
17};
18
19// Case interface
20// This is not possible with interface
21interface ButtonProps {
22 label: string;
23 onClick: () => void;
24 variant: 'primary' | 'secondary'; // Error: String literal types are not allowed in interfaces
25}
8. Intersection Types in React component
When you need to combine multiple types, the type is more flexible.
1type BaseProps = { id: string; };
2type AdvancedProps = { isActive: boolean; };
3
4type CombinedProps = BaseProps & AdvancedProps;
5
6const Component: React.FC<CombinedProps> = ({ id, isActive }) => {
7 return (
8 <div
9 id={id}
10 className={isActive ? 'active' : ''}
11 >
12 Content
13 </div>
14 );
15}
9. Tuples in React component
When you need to define a tuple type, type is the only option.
1type TupleProps = { coordinates: [number, number]; };
2
3const CoordinateDisplay: React.FC<TupleProps> = ({ coordinates }) => {
4 return (
5 <div>
6 X: {coordinates[0]}, Y: {coordinates[1]}
7 </div>
8 );
9};
Using interface (Not Possible)
10. Mapped Types in React component
When you need to create a mapped type, type is more suitable.
1type ReadonlyProps<T> = { readonly [P in keyof T]: T[P]; };
2
3type UserProps = { name: string; age: number; };
4
5type ReadonlyUserProps = ReadonlyProps<UserProps>;
6
7const UserComponent: React.FC<ReadonlyUserProps> = ({ name, age }) => {
8 return (
9 <div>
10 Name: {name}, Age: {age}
11 </div>
12 );
13}
Using interface (Not Possible)
1// This is not possible with interface
2interface ReadonlyProps<T> {
3 readonly [P in keyof T]: T[P]; // Error: Mapped types are not allowed in interfaces
4}
Conclusion
While interface is useful for defining object shapes that are intended to be extended or implemented by classes, type offers more versatility and power for defining complex types. By understanding the type's strengths, you can make more informed decisions in your TypeScript projects.
In summary, use type when you need:
This flexibility makes type a better choice in many scenarios.